mirror of
https://github.com/WordPress/five-for-the-future.git
synced 2025-07-02 09:11:17 +03:00
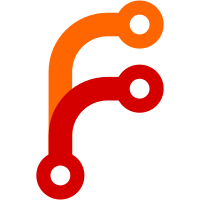
Contributors associated with pledges have a state: they can be confirmed or unconfirmed. They also have some important meta data, namely when they were confirmed. Thus, managing contributor data for pledges is more robust if we treat them as their own post type instead of as a multidimensional array of post meta data. This also reorganizes some of the functions related to pledges so that things are more consistent between the pledge CPT and the contributior CPT. Fixes #11
178 lines
5.3 KiB
PHP
178 lines
5.3 KiB
PHP
<?php
|
|
namespace WordPressDotOrg\FiveForTheFuture\Contributor;
|
|
|
|
use WordPressDotOrg\FiveForTheFuture;
|
|
use WordPressDotOrg\FiveForTheFuture\Pledge;
|
|
use WP_Error, WP_Post;
|
|
|
|
defined( 'WPINC' ) || die();
|
|
|
|
const SLUG = 'contributor';
|
|
const SLUG_PL = 'contributors';
|
|
const CPT_ID = FiveForTheFuture\PREFIX . '_' . SLUG;
|
|
|
|
add_action( 'init', __NAMESPACE__ . '\register_custom_post_type', 0 );
|
|
add_filter( 'manage_edit-' . CPT_ID . '_columns', __NAMESPACE__ . '\add_list_table_columns' );
|
|
add_action( 'manage_' . CPT_ID . '_posts_custom_column', __NAMESPACE__ . '\populate_list_table_columns', 10, 2 );
|
|
|
|
/**
|
|
* Register the post type(s).
|
|
*
|
|
* @return void
|
|
*/
|
|
function register_custom_post_type() {
|
|
$labels = array(
|
|
'name' => _x( 'Contributors', 'Pledges General Name', 'wporg' ),
|
|
'singular_name' => _x( 'Contributor', 'Pledge Singular Name', 'wporg' ),
|
|
'menu_name' => __( 'Five for the Future', 'wporg' ),
|
|
'archives' => __( 'Contributor Archives', 'wporg' ),
|
|
'attributes' => __( 'Contributor Attributes', 'wporg' ),
|
|
'parent_item_colon' => __( 'Parent Contributor:', 'wporg' ),
|
|
'all_items' => __( 'Contributors', 'wporg' ),
|
|
'add_new_item' => __( 'Add New Contributor', 'wporg' ),
|
|
'add_new' => __( 'Add New', 'wporg' ),
|
|
'new_item' => __( 'New Contributor', 'wporg' ),
|
|
'edit_item' => __( 'Edit Contributor', 'wporg' ),
|
|
'update_item' => __( 'Update Contributor', 'wporg' ),
|
|
'view_item' => __( 'View Contributor', 'wporg' ),
|
|
'view_items' => __( 'View Contributors', 'wporg' ),
|
|
'search_items' => __( 'Search Contributors', 'wporg' ),
|
|
'not_found' => __( 'Not found', 'wporg' ),
|
|
'not_found_in_trash' => __( 'Not found in Trash', 'wporg' ),
|
|
'insert_into_item' => __( 'Insert into contributor', 'wporg' ),
|
|
'uploaded_to_this_item' => __( 'Uploaded to this contributor', 'wporg' ),
|
|
'items_list' => __( 'Contributors list', 'wporg' ),
|
|
'items_list_navigation' => __( 'Contributors list navigation', 'wporg' ),
|
|
'filter_items_list' => __( 'Filter contributors list', 'wporg' ),
|
|
);
|
|
|
|
$args = array(
|
|
'labels' => $labels,
|
|
'supports' => array( 'title' ),
|
|
'hierarchical' => false,
|
|
'public' => false,
|
|
'show_ui' => true,
|
|
'show_in_menu' => 'edit.php?post_type=' . Pledge\CPT_ID,
|
|
'menu_position' => 25,
|
|
'show_in_admin_bar' => false,
|
|
'show_in_nav_menus' => false,
|
|
'can_export' => false,
|
|
'taxonomies' => array(),
|
|
'has_archive' => false,
|
|
'exclude_from_search' => true,
|
|
'publicly_queryable' => false,
|
|
'capability_type' => 'page',
|
|
'show_in_rest' => false, // todo Maybe turn this on later.
|
|
);
|
|
|
|
register_post_type( CPT_ID, $args );
|
|
}
|
|
|
|
/**
|
|
* Add columns to the Contributors list table.
|
|
*
|
|
* @param array $columns
|
|
*
|
|
* @return array
|
|
*/
|
|
function add_list_table_columns( $columns ) {
|
|
$first = array_slice( $columns, 0, 2, true );
|
|
$last = array_slice( $columns, 2, null, true );
|
|
|
|
$new_columns = array(
|
|
'pledge' => __( 'Pledge', 'wporg' ),
|
|
);
|
|
|
|
return array_merge( $first, $new_columns, $last );
|
|
}
|
|
|
|
/**
|
|
* Render content in the custom columns added to the Contributors list table.
|
|
*
|
|
* @param string $column
|
|
* @param int $post_id
|
|
*
|
|
* @return void
|
|
*/
|
|
function populate_list_table_columns( $column, $post_id ) {
|
|
switch ( $column ) {
|
|
case 'pledge':
|
|
$contributor = get_post( $post_id );
|
|
$pledge = get_post( $contributor->post_parent );
|
|
|
|
$pledge_name = get_the_title( $pledge );
|
|
|
|
if ( current_user_can( 'edit_post', $pledge->ID ) ) {
|
|
$pledge_name = sprintf(
|
|
'<a href="%1$s">%2$s</a>',
|
|
get_edit_post_link( $pledge ),
|
|
$pledge_name
|
|
);
|
|
}
|
|
|
|
echo $pledge_name;
|
|
break;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Create a contributor post as a child of a pledge post.
|
|
*
|
|
* @param string $wporg_username
|
|
* @param int $pledge_id
|
|
*
|
|
* @return int|WP_Error Post ID on success. Otherwise WP_Error.
|
|
*/
|
|
function create_new_contributor( $wporg_username, $pledge_id ) {
|
|
$args = array(
|
|
'post_type' => CPT_ID,
|
|
'post_title' => sanitize_user( $wporg_username ),
|
|
'post_parent' => $pledge_id,
|
|
'post_status' => 'pending',
|
|
);
|
|
|
|
return wp_insert_post( $args, true );
|
|
}
|
|
|
|
/**
|
|
* Get the contributor posts associated with a particular pledge post.
|
|
*
|
|
* @param int $pledge_id The post ID of the pledge.
|
|
* @param string $status Optional. 'all', 'pending', or 'publish'.
|
|
*
|
|
* @return array An array of contributor posts. If $status is set to 'all', will be
|
|
* a multidimensional array with keys for each status.
|
|
*/
|
|
function get_pledge_contributors( $pledge_id, $status = 'publish' ) {
|
|
$args = array(
|
|
'post_type' => CPT_ID,
|
|
'post_parent' => $pledge_id,
|
|
'numberposts' => -1,
|
|
'orderby' => 'title',
|
|
'order' => 'asc',
|
|
);
|
|
|
|
if ( 'all' === $status ) {
|
|
$args['post_status'] = array( 'pending', 'publish' );
|
|
} else {
|
|
$args['post_status'] = sanitize_key( $status );
|
|
}
|
|
|
|
$posts = get_posts( $args );
|
|
|
|
if ( 'all' === $status && ! empty( $posts ) ) {
|
|
$initial = array(
|
|
'publish' => array(),
|
|
'pending' => array(),
|
|
);
|
|
|
|
$posts = array_reduce( $posts, function( $carry, WP_Post $item ) {
|
|
$carry[ $item->post_status ][] = $item;
|
|
|
|
return $carry;
|
|
}, $initial );
|
|
}
|
|
|
|
return $posts;
|
|
}
|